To be fair, the documentation for Jersey does include a section on filter and interceptor execution order. If you like reading a wall of text and want to know the absolute maximum test case, that's the place to go. Not all of the steps described there apply in all cases, which is why I think my simple case-by-case breakdown is going to be useful.
First lets look at some code. I'll make the full source available at the conclusion of this series of articles so I'm only showing the bare minimum required code at the moment.
The application class extends ResourceConfig and disables automatic feature discovery (because I want to control exactly what is loaded).
Java
public class JerseyServiceRSApplication extends ResourceConfig {
public JerseyServiceRSApplication() {
property(CommonProperties.FEATURE_AUTO_DISCOVERY_DISABLE, true);
register(MyWriterInterceptor.class);
register(MyResponseFilter.class);
register(MyRequestFilter.class);
register(MyPreMatchingRequestFilter.class);
register(MyResource.class);
}
}
Then come the filters, intercepts and the resource class itself. The implementations of filters and interceptors do not do much apart from logging, they are for demonstrative purposes after all. The resource is set to respond to a GET request on the /hello/{name} path and returns a string that says "Hello {name}" as the response.
Java
@PreMatching
public class MyPreMatchingRequestFilter implements ContainerRequestFilter {
@Override
public void filter(ContainerRequestContext crc)
{
Logger.getLogger("net.igorkromin.Logger")
.debug(">>> MyPreMatchingRequestFilter");
}
}
@Provider
public class MyRequestFilter implements ContainerRequestFilter {
@Override
public void filter(ContainerRequestContext crc)
{
Logger.getLogger("net.igorkromin.Logger")
.debug(">>> MyRequestFilter");
}
}
public class MyResponseFilter implements ContainerResponseFilter {
@Override
public void filter(ContainerRequestContext requestContext,
ContainerResponseContext responseContext)
throws IOException
{
Logger.getLogger("net.igorkromin.Logger")
.debug(">>> MyResponseFilter");
}
}
public class MyWriterInterceptor implements WriterInterceptor {
@Override
public void aroundWriteTo(WriterInterceptorContext context)
throws IOException, WebApplicationException
{
Logger.getLogger("net.igorkromin.Logger")
.debug(">>> MyWriterInterceptor");
context.proceed();
}
}
@Path("/hello")
@Produces({ "text/plain" })
public class MyResource {
@GET
@Path("/{name}")
public String sayHelloTo(@PathParam("name") String name)
{
Logger.getLogger("net.igorkromin.Logger")
.debug(">>> MyResource");
return "Hello " + name;
}
}
That's quite straight forward. Once deployed the application will accept requests on the <service_uri>/hello/{name} endpoint.
Lets see what happens after this cURL request...
cURL
curl -v http://myserver:8001/JerseyServiceRS/Service/hello/test
The response is, as expected...
Response
< HTTP/1.1 200 OK
< Date: Thu, 19 Oct 2017 07:18:02 GMT
< Content-Length: 10
< Content-Type: text/plain
< X-Powered-By: Servlet/3.0 JSP/2.2
<
Hello test
After looking at the logs to confirm, this is the execution order of the filters and interceptors...
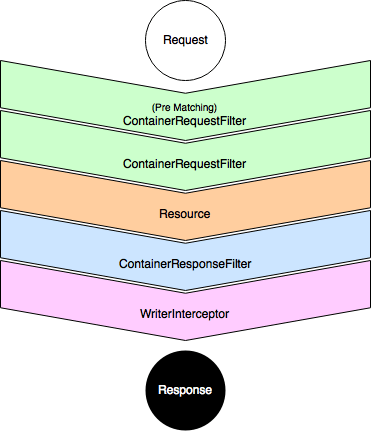
The order is quite trivial, pre-matching filter is run first, followed by the post-matching filter, then the resource class is executed, followed by the response filter and finally the writer interceptor.
Now what if we tried to request something that doesn't map to a resource? Lets see...
cURL
curl -v http://myserver:8001/JerseyServiceRS/Service/hello2/test
Since the <service_uri>/hello2/{name} endpoint doesn't exist the response is...
Response
< HTTP/1.1 404 Not Found
< Date: Thu, 19 Oct 2017 07:18:40 GMT
< Content-Length: 9
< Content-Type: text/html; charset=UTF-8
< X-Powered-By: Servlet/3.0 JSP/2.2
<
Not Found
You may expect that none of the classes in your application were called, but that's not correct. According to the logs, the execution order was...
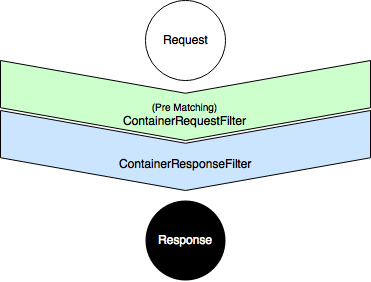
That's right the pre-matching request filter is executed because Jersey invokes it before it tries to resolve a valid resource path inside your application. The response filter is also invoked but interestingly the writer interceptor is not, this is because the default error handling follows a slightly different code path in Jersey.
The GET case is quite trivial but shows some important behaviours in Jersey. I'll be following up with more advanced topics covering POST requests and message body readers/writers, exception handlers, etc. Keep an eye out for future posts, I'll link them here as they are available.
Update (02 November 2017) - The next article in this series is available here - Jersey JAX-RS filters and interceptors execution order for a POST request.
-i