Update: There is an issue with AdSense backed ad slots right now that causes the containing page to scroll down to the ad slot as soon as refresh() is called. I started a discussion topic on it on the support forums here. Unfortunately this 'bug' makes infinite scroll pages virtually unusable right now.
Please read this article before you go further: Google DFP with AdSense fallback is causing infinite scroll pages to go haywire.
Update: Looks like Google have fixed this problem as of 22 March 2017.
The trick is to use Google's DoubleClick for Publishers or DFP for short. DFP is an ad server, whereas AdSense is an ad network. The major difference there is with an ad server you have full control over how ads are served, where they are placed and how you want to manage them. Typically you would use this to sell ads on your site, but you can also instruct DFP to fall back to AdSense. This gives you a lot of flexibility in how ads are delivered and where they are placed as well as optionally being able to refresh them periodically!
Hold on though, it gets even better. If you have an AdSense account, you automatically get a DFP account! There is also extensive documentation on how to implement DFP, with advanced examples that cover infinite scroll pages, ad refreshing and responsive ads.
My example builds on the example that Google already provides, and adds screenshots to make it easier to follow.
First you have to set up an Ad Unit. On the DFP 'Inventory' tab, go to 'Ad units' and click 'New ad unit'.
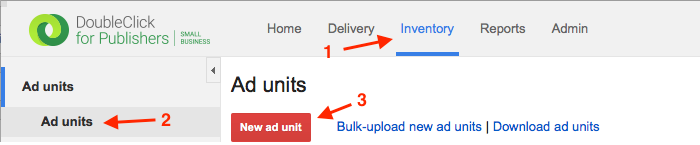
Next you need to enter a code for this Ad Unit, in my case I use 'MyAdsenseAds' as an example, and then I override the AdSense settings to enable the maximize revenue option.
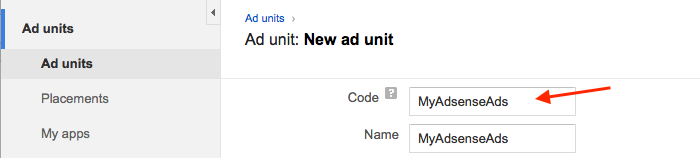
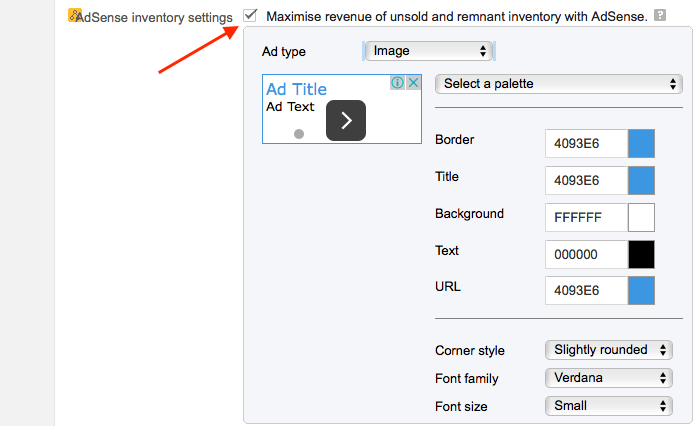
Take note of the Ad Unit Code you entered as that's needed later. Next you need to get the Network Code (publisher ID). This is done from the 'Admin' tab in 'All network settings'.
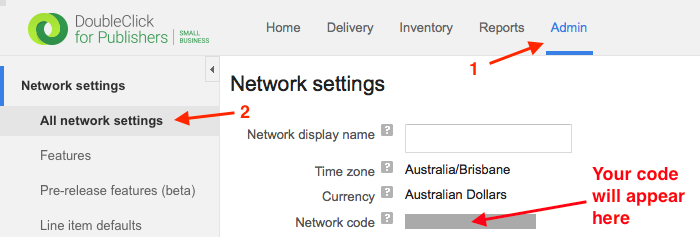
So lets see how this is implemented in code. First the standard DFP code is required, this should be added to the <head> element of your web site inside a <script> tag.
JavaScript
var googletag = googletag || {};
googletag.cmd = googletag.cmd || [];
(function() {
var gads = document.createElement('script');
gads.async = true;
gads.type = 'text/javascript';
var useSSL = 'https:' == document.location.protocol;
gads.src = (useSSL ? 'https:' : 'http:') +
'//www.googletagservices.com/tag/js/gpt.js';
var node = document.getElementsByTagName('script')[0];
node.parentNode.insertBefore(gads, node);
})();
Next you need to add some initialisation code. This should run after the page is fully loaded, so in my case I use jQuery's $(document).ready() function and have it run the following...
JavaScript
googletag.cmd.push(function() {
googletag.pubads().enableSingleRequest();
googletag.pubads().disableInitialLoad();
googletag.enableServices();
});
That code sets up DFP but doesn't load any ads yet.
Next I have a DIV element inside my HTML that is hidden by default, this should be placed right before the closing </body> tag. That element is used as a template and contains the DIV that will eventually hold the ad.
HTML
<div style="display: none;" id="tb_gpt_ad"><div class="tb_gpt_ad"></div></div>
Elsewhere in my JavaScript code I keep track of these global variables. I will leave that code out of this example since that's implementation specific, but these variables are used further down so make sure they are actually defined.
JavaScript
/* unique ID for an ad unit, can simply be incremented each time an ad unit is created */
var adId;
/* Network Code / Publisher ID */
var dfpPublisherId;
/* Ad Unit Code */
var dfpAdUnitCode
Since this example is about infinite scroll pages, I will assume that your code is already handling inserting of new content and hence knows where in the DOM it is. With that in mind, I am going to use the insertAfterEl variable to specify where in the DOM the new ad unit should be inserted after. This variable is a jQuery element.
So this is what my ad insertion code looks like...
JavaScript
var slotId = 'tb_gpt_ad_' + adId;
/* clone and display ad container element */
var newAd = $('#tb_gpt_ad').clone().insertAfter(insertAfterEl).show();
/* id of the inner div has to be unique since DFP code looks for this element */
newAd.find('.tb_gpt_ad').attr('id', slotId);
/* create and fetch the ad from DFP server */
googletag.cmd.push(function() {
var slot = googletag.defineSlot('/' + dfpPublisherId + '/' + dfpAdUnitCode,
[728, 90], slotId).addService(googletag.pubads());
googletag.display(slotId);
googletag.pubads().refresh([slot]);
});
That's all there is to it. I wrap this in a function of course and call that function wherever I want to insert an Ad dynamically into the DOM. In the above example I use a static size of 728x90, this can be changed to whatever sizes you have defined for your Ad Unit in DFP. This size must match one of the sizes you define in DFP, so be sure you have the correct ad unit dimensions selected when creating the ad unit.
If the above is not clear, I put together a full example of an infinite scroll page as well an example of an infinite scroll page with Google DFP. You will need to update the code and put in your publisher ID and ad unit code for the ads to come up, but apart from that the ads will be requested every 5th content block.
If you need to use DFP on responsive pages check out this article I've written. I've also wrote another article on how to Insert Google DFP ads with Backbone, Underscore and jQuery.
-i